Using multiplatform resources in your app
Edit pageLast modified: 20 March 2025When you've set up the resources for your project, build the project to generate the special Res
class which provides access to resources. To regenerate the Res
class and all the resource accessors, build the project again or re-import the project in the IDE.
After that, you can use the generated class to access the configured multiplatform resources from your code or from external libraries.
Importing the generated class
To use the prepared resources, import the generated class, for example:
import project.composeapp.generated.resources.Res
import project.composeapp.generated.resources.example_image
Here:
project
is the name of your projectcomposeapp
is the module where you placed the resource directoriesRes
is the default name for the generated classexample_image
is the name of an image file in thecomposeResources/drawable
directory (example_image.png
, for example).
Customizing accessor class generation
You can customize the generated Res
class to suit your needs using Gradle settings.
In the compose.resources {}
block of the build.gradle.kts
file, you can specify several settings that affect the way the Res
class is generated for your project. An example configuration looks like this:
compose.resources {
publicResClass = false
packageOfResClass = "me.sample.library.resources"
generateResClass = auto
}
publicResClass
set totrue
makes the generatedRes
class public. By default, the generated class is internal.packageOfResClass
allows you to assign the generatedRes
class to a particular package (to access within the code, as well as for isolation in a final artifact). By default, Compose Multiplatform assigns the{group name}.{module name}.generated.resources
package to the class.generateResClass
set toalways
makes the project unconditionally generate theRes
class. This may be useful when the resource library is only available transitively. By default, Compose Multiplatform uses theauto
value to generate theRes
class only if the current project has an explicitimplementation
orapi
dependency on the resource library.
Resource usage
Images
You can access drawable resources as simple images, rasterized images or XML vectors. SVG images are supported on all platforms except Android.
To access drawable resources as
Painter
images, use thepainterResource()
function:@Composable fun painterResource(resource: DrawableResource): Painter {...}
The
painterResource()
function takes a resource path and returns aPainter
value. The function works synchronously on all targets except for web. For the web target, it returns an emptyPainter
for the first recomposition that is replaced with the loaded image in subsequent recompositions.painterResource()
loads either aBitmapPainter
for rasterized image formats, such as.png
,.jpg
,.bmp
,.webp
, or aVectorPainter
for the Android XML vector drawable format.XML vector drawables have the same format as Android, except that they don't support external references to Android resources.
To access drawable resources as an
ImageBitmap
rasterized image, use theimageResource()
function:@Composable fun imageResource(resource: DrawableResource): ImageBitmap {...}
To access drawable resources as an
ImageVector
XML vector, use thevectorResource()
function:@Composable fun vectorResource(resource: DrawableResource): ImageVector {...}
Here's an example of how you can access images in your Compose Multiplatform code:
Image(
painter = painterResource(Res.drawable.my_icon),
contentDescription = null
)
Strings
Store all string resources in XML files in composeResources/values
directories. A static accessor is generated for each item in each file.
Simple strings
To store a simple string, add a <string>
element to your XML:
<resources>
<string name="app_name">My awesome app</string>
<string name="title">Some title</string>
</resources>
To get string resources as a String
, use the following code:
@Composable
fun stringResource(resource: StringResource): String {...}
@Composable
fun stringResource(resource: StringResource, vararg formatArgs: Any): String {...}
For example:
Text(stringResource(Res.string.app_name))
suspend fun getString(resource: StringResource): String
suspend fun getString(resource: StringResource, vararg formatArgs: Any): String
For example:
coroutineScope.launch {
val appName = getString(Res.string.app_name)
}
You can use special symbols in string resources:
\n
— for a new line\t
— for a tab symbol\uXXXX
— for a specific Unicode character
You don't need to escape special XML characters like "@" or "?" as you do for Android strings.
String templates
Currently, arguments have basic support for string resources. When creating a template, use the %<number>
format to place arguments within the string and include a $d
or $s
suffix to indicate that it is a variable placeholder and not simple text. For example:
<resources>
<string name="str_template">Hello, %2$s! You have %1$d new messages.</string>
</resources>
After creating and importing the string template resource, you can refer to it while passing the arguments for placeholders in the correct order:
Text(stringResource(Res.string.str_template, 100, "User_name"))
There is no difference between the $s
and $d
suffixes, and no others are supported. You can put the %1$s
placeholder in the resource string and use it to display a fractional number, for example:
Text(stringResource(Res.string.str_template, "User_name", 100.1f))
String arrays
You can group related strings into an array and automatically access them as a List<String>
object:
<resources>
<string name="app_name">My awesome app</string>
<string name="title">Some title</string>
<string-array name="str_arr">
<item>item \u2605</item>
<item>item \u2318</item>
<item>item \u00BD</item>
</string-array>
</resources>
To get the corresponding list, use the following code:
@Composable
fun stringArrayResource(resource: StringArrayResource): List<String> {...}
For example:
Text(stringArrayResource(Res.array.str_arr)[0])
suspend fun getStringArray(resource: StringArrayResource): List<String>
For example:
coroutineScope.launch {
val appName = getStringArray(Res.array.str_arr)
}
Plurals
When your UI displays quantities of something, you might want to support grammatical agreement for different numbers of the same thing (one book, many books, and so on) without creating programmatically unrelated strings.
The concept and base implementation in Compose Multiplatform are the same as for quantity strings on Android. See the Android documentation for more about best practices and nuances of using plurals in your project.
The supported variants are
zero
,one
,two
,few
,many
, andother
. Note that not all variants are even considered for every language: for example,zero
is ignored for English because it is the same as any other plural except 1. Rely on a language specialist to know what distinctions the language actually insists upon.It's often possible to avoid quantity strings by using quantity-neutral formulations such as "Books: 1". If this doesn't worsen the user experience,
To define a plural, add a <plurals>
element to any .xml
file in your composeResources/values
directory. A plurals
collection is a simple resource referenced using the name attribute (not the name of the XML file). As such, you can combine plurals
resources with other simple resources in one XML file under one <resources>
element:
<resources>
<string name="app_name">My awesome app</string>
<string name="title">Some title</string>
<plurals name="new_message">
<item quantity="one">%1$d new message</item>
<item quantity="other">%1$d new messages</item>
</plurals>
</resources>
To access a plural as a String
, use the following code:
@Composable
fun pluralStringResource(resource: PluralStringResource, quantity: Int): String {...}
@Composable
fun pluralStringResource(resource: PluralStringResource, quantity: Int, vararg formatArgs: Any): String {...}
For example:
Text(pluralStringResource(Res.plurals.new_message, 1, 1))
suspend fun getPluralString(resource: PluralStringResource, quantity: Int): String
suspend fun getPluralString(resource: PluralStringResource, quantity: Int, vararg formatArgs: Any): String
For example:
coroutineScope.launch {
val appName = getPluralString(Res.plurals.new_message, 1, 1)
}
Fonts
Store custom fonts in the composeResources/font
directory as *.ttf
or *.otf
files.
To load a font as a Font
type, use the Font()
function:
@Composable
fun Font(
resource: FontResource,
weight: FontWeight = FontWeight.Normal,
style: FontStyle = FontStyle.Normal
): Font
For example:
val fontAwesome = FontFamily(Font(Res.font.font_awesome))
Raw files
To load any raw file as a byte array, use the Res.readBytes(path)
function:
suspend fun readBytes(path: String): ByteArray
You can place raw files in the composeResources/files
directory and create any hierarchy inside it.
For example, to access raw files, use the following code:
var bytes by remember {
mutableStateOf(ByteArray(0))
}
LaunchedEffect(Unit) {
bytes = Res.readBytes("files/myDir/someFile.bin")
}
Text(bytes.decodeToString())
coroutineScope.launch {
val bytes = Res.readBytes("files/myDir/someFile.bin")
}
Convert byte arrays into images
If the file you are reading is a bitmap (JPEG, PNG, BMP, WEBP) or an XML vector image, you can use the following functions to convert them into ImageBitmap
or ImageVector
objects suitable for the Image()
composable.
Access the raw files as shown in the Raw files section, then pass the result to a composable:
// bytes = Res.readBytes("files/example.png")
Image(bytes.decodeToImageBitmap(), null)
// bytes = Res.readBytes("files/example.xml")
Image(bytes.decodeToImageVector(LocalDensity.current), null)
On every platform except Android, you can also turn an SVG file into a Painter
object:
// bytes = Res.readBytes("files/example.svg")
Image(bytes.decodeToSvgPainter(LocalDensity.current), null)
Generated maps for resources and string IDs
For ease of access, Compose Multiplatform also maps resources with string IDs. You can access them by using the filename as the key:
val Res.allDrawableResources: Map<String, DrawableResource>
val Res.allStringResources: Map<String, StringResource>
val Res.allStringArrayResources: Map<String, StringArrayResource>
val Res.allPluralStringResources: Map<String, PluralStringResource>
val Res.allFontResources: Map<String, FontResource>
An example of passing a mapped resource to a composable:
Image(painterResource(Res.allDrawableResources["compose_multiplatform"]!!), null)
Compose Multiplatform resources as Android assets
Starting with Compose Multiplatform 1.7.3, all multiplatform resources are packed into Android assets. This enables Android Studio to generate previews for Compose Multiplatform composables in Android source sets.
warning
Android Studio previews are available only for composables in an Android source set. They also require one of the latest versions of AGP: 8.5.2, 8.6.0-rc01, or 8.7.0-alpha04.
Using Multiplatform resources as Android assets also makes possible direct access from WebViews and media player components on Android, since resources can be reached by a simple path, for example Res.getUri("files/index.html")
.
An example of an Android composable displaying a resource HTML page with a link to a resource image:
AndroidView(factory = { WebView(it).apply
{...}
The example works with this simple HTML file:
<title>Cat Resource</title>
{...}
Both resource files in this example are located in the commonMain
source set:
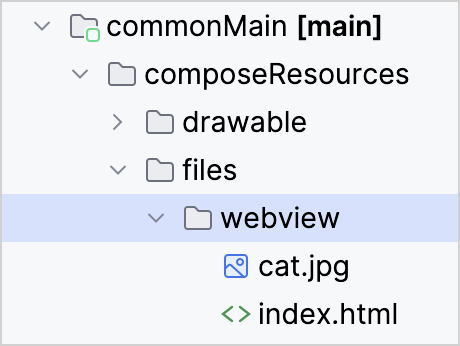
Preloading of resources for web targets
The web resources like fonts and images are loaded asynchronously using the fetch
API. During the initial load or with slower network connections, resource fetching can cause visual glitches, such as FOUT or displaying placeholders instead of images.
A typical example of this issue is when a Text()
component contains text in a custom font, but the font with the necessary glyphs is still loading. In this case, users may temporarily see the text in default font or even empty boxes and question marks instead of characters. Similarly, for images or drawables, users may observe a placeholder like a blank or black box until the resource is fully loaded.
To prevent visual glitches, you can use built-in browser features for preloading of resources, the Compose Multiplatform preload API, or a combination of both.
Preload resources using browser features
In modern browsers, you can preload resources using the <link>
tag with the rel="preload"
attribute. This attribute instructs the browser to prioritize downloading and caching resources like fonts and images before the application starts, ensuring that these resources are available early.
For example, to enable in-browser preloading of a font:
Build your application's web distribution:
./gradlew :composeApp:wasmJsBrowserDistribution
Find the required resource in the generated
dist
directory and save the path.Open the
wasmJsMain/resources/index.html
file and add a<link>
tag inside the<head>
element.Set the
href
attribute to the resource path:
<link rel="preload" href="./composeResources/username.composeapp.generated.resources/font/FiraMono-Regular.ttf" as="fetch" type="font/ttf" crossorigin/>
Preload resources using the Compose Multiplatform preload API
Even if you preloaded the resources in the browser, they are cached as raw bytes that still need to be converted into a format suitable for rendering, such as FontResource
and DrawableResource
. When the application requests the resource for the first time, the conversion is done asynchronously, which may again result in flickering. To further optimize the experience, Compose Multiplatform resources have their own internal cache for higher-level representations of the resources, that can also be preloaded.
Compose Multiplatform 1.8.0-rc01 introduces an experimental API for preloading font and image resources on web targets: preloadFont()
, preloadImageBitmap()
, and preloadImageVector()
.
Additionally, you can set fallback fonts different from the default bundled option if you require special characters like emojis. To specify a fallback font, use the FontFamily.Resolver.preload()
method.
The following example demonstrates how to use preloading and a fallback font:
fontFamilyResolver.preload(FontFamily(listOf(emojiFont)))
{...}
Interaction with other libraries and resources
Accessing multiplatform resources from external libraries
If you want to process multiplatform resources using other libraries included in your project, you can pass platform-specific file paths to these other APIs. To get a platform-specific path, call the Res.getUri()
function with the project path to the resource:
val uri = Res.getUri("files/my_video.mp4")
Now that the uri
variable contains the absolute path to the file, any external library can use that path to access the file in a manner that suits it.
For Android-specific uses, multiplatform resources are also packed as Android assets.
Remote files
In the context of the resource library, only files that are part of the application are considered resources.
You can load remote files from the internet using their URL using specialized libraries:
Using Java resources
While you can use Java resources with Compose Multiplatform, they don't benefit from extended features provided by the framework: generated accessors, multimodule support, localization, and so on. Consider transitioning fully to the multiplatform resource library to unlock that potential.
With Compose Multiplatform 1.7.3, the resources API available in the compose.ui
package is deprecated. If you still need to work with Java resources, copy the following implementation to your project to ensure that your code works after you upgrade to Compose Multiplatform 1.7.0 or above:
internal fun painterResource(resourcePath: String): Painter
{...}
What's next?
Check out the official demo project that shows how resources can be handled in a Compose Multiplatform project targeting iOS, Android, and desktop.