Getting started with C++
This tutorial gets you up to speed with C++ development in JetBrains Fleet. It covers the installation, project setup, and working with code.
Install Fleet
Install JetBrains Toolbox 1.22.10970 or later: the Download page.
Download and install JetBrains Toolbox.
In JetBrains Toolbox, click Install near the JetBrains Fleet icon.
Set up a workspace
Prepare a compilation database for your project
JetBrains Fleet supports C++ projects with compilation databases.
A compilation database is a JSON file named compile_commands.json, which contains structured data about every compilation unit in the project.
Follow this instruction to generate a compilation database for your project.
Open a project
Press ⌘ O or select File | Open from the menu.
Select your project root folder and click Open:
The opened directory becomes the root of your workspace:
Switch to Smart Mode
Enable Smart Mode
Smart Mode turns on code insight features for your C++ code.
Click the Smart Mode Status icon in the top-right corner. In the popup that appears, click Enable.
Troubleshooting Smart Mode: LSP can't start
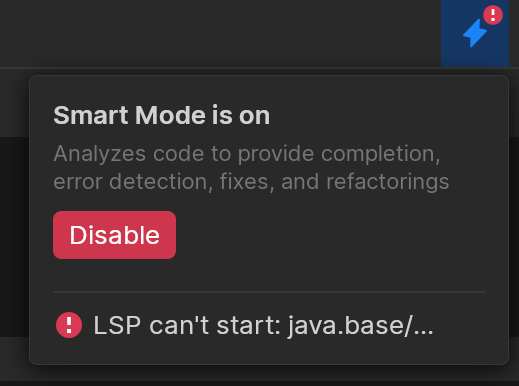
This message indicates that Fleet might not have been able to download Clangd because of a firewall. Follow the steps below for a workaround.
Download Clangd using the following links:
Put the downloaded file into the ~/.fleet/ directory.
Restart Fleet.
Here's what you can do in Smart Mode. The below is not an exhaustive list of Smart Mode features, rather a couple of examples that'll help you to get the feel of how it works in Fleet.
Spot syntax errors
All the errors in your code are highlighted red:
Complete code automatically
Context-aware completion gives a list of suggestions for names, types, and keywords:
Among the completion suggestions, you can find templates for common code constructs. Select one from the list, and then use Tab to move the caret between stubs:
Available code snippets:
typedef [type] [name];
using [name] [type];
namespace [name] = [namespace];
using namespace [identifier];
using [qualifier]::[name];
using typename [qualifier]::[name];
template<class [name]>
template<typename [name]>
switch ([condition]) { [cases] }
case [expression]:
goto [label];
dynamic_cast<[type]>([expression])
static_cast<[type]>([expression])
reinterpret_cast<[type]>([expression])
const_cast<[type]>([expression])
catch ([declaration]) { [statements] }
concept [name] = [constraint];
#define [macro]([args])
Reduce caret jumps
To transform an already typed expression into a different one without moving the caret, add a dot at the end. You will see postfix completion options among the list of suggestions:
For example, you can choose if, and the initial expression will get wrapped into an
if
statement:
View parameter information
Press ⌘ I to get the list of parameter names in a function call:
Click Show all to view all the usages.
Check documentation in a popup
Hover over a class, function, field, or another element to see where and how it is defined:
Rename code items
Select an item to be renamed, press ⌃ R, and enter the new name:
Navigate the codebase
Navigate to the declaration of a symbol with ⌘ B.
Find usages of a symbol with ⌘ U:
Skim over the errors with ⌘ E and ⌘ ⇧ E.
Build and run your program
Fleet does not provide automation for the process of building a running C/C++ applications.
However, you can use Fleet's language-agnostic run configurations for that. Find below an example for the case of CMake: