Kotlin
Parameter hints
Controls whether hints should appear for function and constructor arguments.
data class User(val id: Int)
fun printSum(a: Int, b: Int) = println(a + b)
fun hintsDemo() {
val u = User(0) // calling constructor
printSum(1, 1) // calling function
}
Use Exclude list to disable parameter inlay hints for specific methods.
Types
Property types | Controls whether hints should appear when assigning a value to a property whose type is not explicitly specified.
class User(_id: Int = 0) {
val id = _id // inferred property type
}
|
Local variable types | Controls whether hints should show the type when a local variable, whose type is not specified, is assigned some value. The type is not shown for constants.
fun returnHello(): String = "Hello"
fun hintsDemo() {
val s = returnHello()
// "s" gets the String type
}
|
Function return types | Controls whether hints should appear for functions with inferred return types.
fun sum(i1: Int, i2: Int) = i1 + i2
// the return type (Int) is inferred
|
Function parameter types | Controls whether hints should appear for lambda parameters without a type.
fun hintsDemo() {
listOf(1, 2, 3).filter { elem -> // parameter with inferred type
elem >= 3
}
}
|
Lambdas
Return expressions | Controls whether hints should appear for multiline lambdas that return a value. This is useful in complex constructs, where it is easy to forget that a lambda is returning something.
fun numbers(): String {
val numbers = StringBuilder()
return with(numbers) {
// "with" structure starts here
for (letter in '0'..'9') {
append(letter)
}
toString() // used as the return value
}
}
|
Implicit receivers and parameters | Controls whether hints should appear for lambdas' implicit receivers.
fun numbers(): String {
val numbers = StringBuilder()
return with(numbers) {
// the implicit receiver is "numbers" (StringBuilder)
for (letter in '0'..'9') {
append(letter)
}
toString()
}
}
|
URL path inlay
Controls whether an inlay hint should appear at URL mappings allowing you to quickly access the related actions.
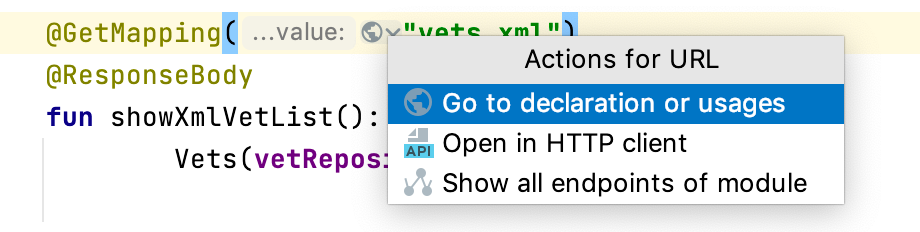
Last modified: 08 March 2021