List of Kotlin live templates
Use live templates to insert common constructs into your code, such as loops, conditions, declarations, or print statements.
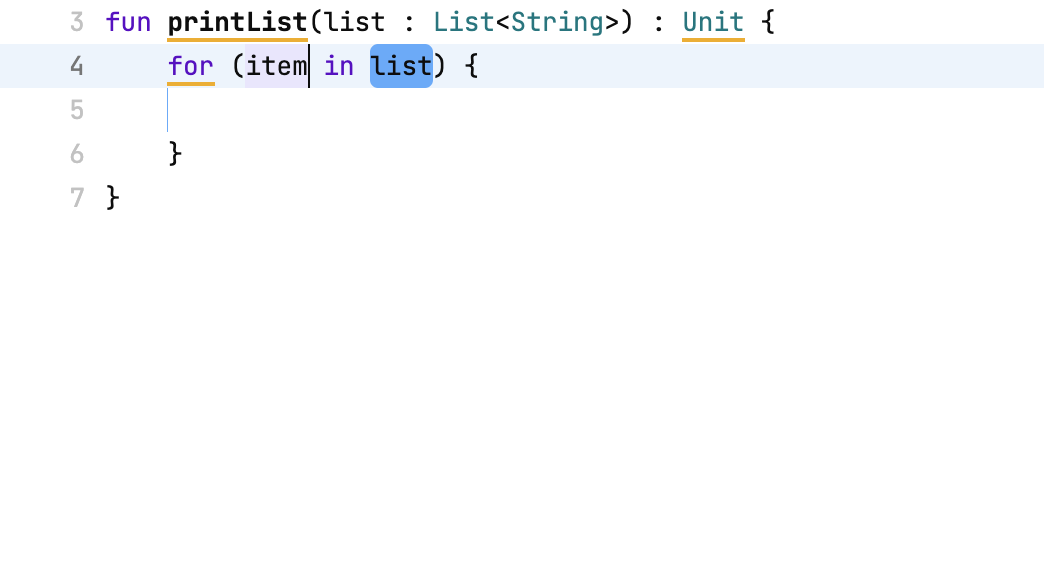
To expand a code snippet, type the corresponding template abbreviation and press ⇥. Keep pressing ⇥ to jump from one variable in the template to the next one. Press ⇧ ⇥ to move to the previous variable.
This table summarizes the live templates that you can use with your Kotlin code.
Abbreviation | Description | Template text | Can be applied in |
---|---|---|---|
anonymous
| Anonymous class |
object : $SUPERTYPE$ {
$END$
}
| Kotlin statement Kotlin expression |
closure
| Closure (function without name) |
{$PARAM$ -> $PARAM_COPY$}
| Kotlin statement Kotlin expression |
exfun
| Extension function |
fun $RECEIVER$.$NAME$($PARAMS$) : $RETURN$ {
$END$
}
| Kotlin class Kotlin top-level |
exval
| Extension read-only property |
val $RECEIVER$.$NAME$ : $TYPE$
get() {
$END$
}
| Kotlin class Kotlin top-level |
exvar
| Extension read-write property |
var $RECEIVER$.$NAME$ : $TYPE$
get() {
$END$
}
set(value) {
}
| Kotlin class Kotlin top-level |
fun0
| Function with no parameters |
fun $NAME$() : $RETURN$ {
$END$
}
| Kotlin statement Kotlin class Kotlin top-level |
fun1
| Function with one parameter |
fun $NAME$($PARAM1$ : $PARAM1TYPE$) : $RETURN$ {
$END$
}
| Kotlin statement Kotlin class Kotlin top-level |
fun2
| Function with two parameters |
fun $NAME$($PARAM1$ : $PARAM1TYPE$, $PARAM2$ : $PARAM2TYPE$) : $RETURN$ {
$END$
}
| Kotlin statement Kotlin class Kotlin top-level |
ifn
| Inserts 'if null' expression |
if ($VAR$ == null) {
$END$
}
| Kotlin expression |
inn
| Inserts 'if not null' expression |
if ($VAR$ != null) {
$END$
}
| Kotlin expression |
interface
| Interface |
interface $NAME$ {
$END$
}
| Kotlin statement Kotlin class Kotlin top-level |
iter
| Iterate over elements of iterable (for-in loop) |
for ($VAR$ in $ITERABLE$) {
$END$
}
| Kotlin statement |
main
| main() function |
fun main($ARGS$) {
$END$
}
| Kotlin top-level |
maina
| main(args) function |
fun main(args: Array<String>) {
$END$
}
| Kotlin top-level |
maino
| main(args) function |
@JvmStatic
fun main(args: Array<String>) {
$END$
}
| Kotlin object declaration |
object
| Anonymous class |
object : $SUPERTYPE$ {
$END$
}
| Kotlin statement Kotlin expression |
psvm
| main() function |
fun main($ARGS$) {
$END$
}
| Kotlin top-level |
psvma
| main(args) function |
fun main(args: Array<String>) {
$END$
}
| Kotlin top-level |
psvmo
| main(args) function |
@JvmStatic
fun main(args: Array<String>) {
$END$
}
| Kotlin object declaration |
serr
| Prints a string to System.err |
System.err.println($END$)
| Kotlin statement |
singleton
| Singleton |
object $NAME$ {
$END$
}
| Kotlin statement Kotlin class Kotlin top-level |
sout
| Prints a string to System.out |
println($END$)
| Kotlin statement |
soutf
| Prints current class and function name to System.out |
println("$CLASS$.$METHOD$")
| Kotlin statement |
soutp
| Prints method parameter names and values to System.out |
println($FORMAT$)
| Kotlin statement |
soutv
| Prints a value to System.out |
println("$EXPR_COPY$ = $DOLLAR${$EXPR$}")
| Kotlin statement |
void
| Function returning nothing |
fun $NAME$($PARAMS$) {
$END$
}
| Kotlin statement Kotlin class Kotlin top-level |