List of Java live templates
Use live templates to insert common constructs into your code, such as loops, conditions, declarations, or print statements.
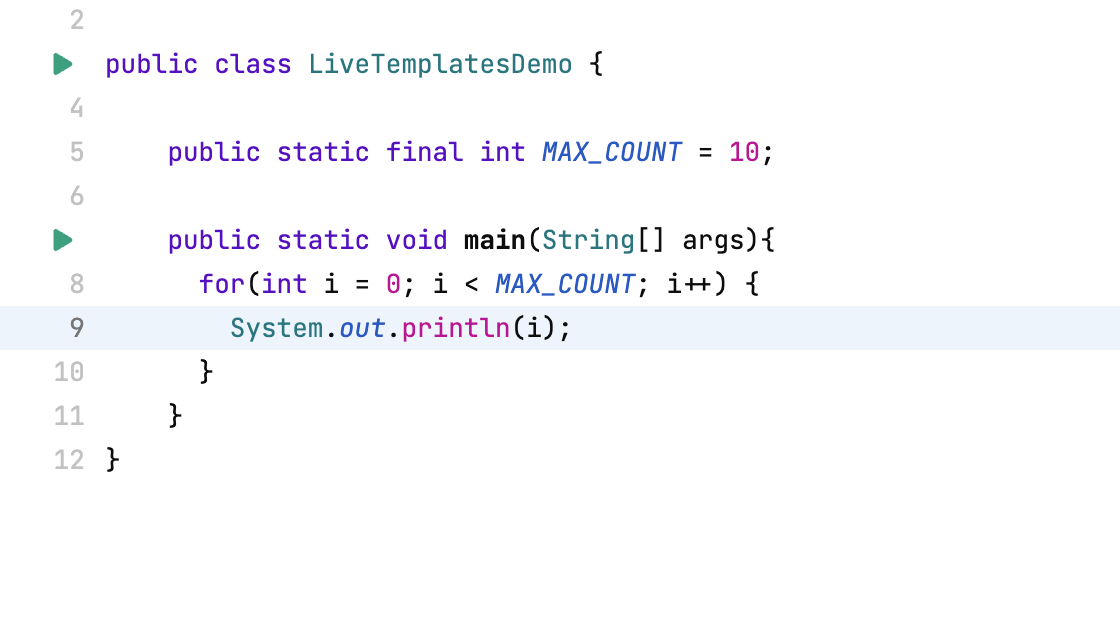
To expand a code snippet, type the corresponding template abbreviation and press ⇥. Keep pressing ⇥ to jump from one variable in the template to the next one. Press ⇧ ⇥ to move to the previous variable.
This table summarizes the live templates that you can use with your Java code.
Live template | Description / code snippet |
---|---|
| Surround with
Callable<Object> callable = new Callable<Object>() {
public Object call() throws Exception {
}
};
|
| Add an
else if() {
}
|
| Create an iteration loop:
for(int i = 0; i < ; i++) {
}
|
| Create a singleton method
public static MyClass getInstance() {
return null;
}
|
| Iterate over the elements of an
for (Object o : ) {
}
(The same as |
| Inserts a null check (an if null statement):
if (list == null) {
}
|
| Inserts a null check (an if not null statement):
if (list != null) {
}
|
| Checks object type with
if ( instanceof ) {
= ()a;
}
|
| Iterates elements of an array:
for(int i = 0; i < array.length; i++) {
= array[i];
}
|
| Iterates over the elements of a
for(Iterator iterator = collection.iterator(); iterator.hasNext(); ) {
Object o = iterator.next();
}
|
| Iterates over the elements of
while(enumeration.hasMoreElements()){
Object nextElement = enumeration.nextElement();
}
|
| Iterates over the elements of an
for (Object o : ) {
}
(The same as |
| Iterates over a
while(iterator.hasNext()){
Object next = iterator.next();
}
|
| Iterates over the elements of a
for (int i = 0; i < list.size(); i++) {
Object o = list.get(i);
}
|
| Iterates over the tokens of a
for (StringTokenizer stringTokenizer = new StringTokenizer(); stringTokenizer.hasMoreTokens(); ) {
String s = stringTokenizer.nextToken();
}
|
| Performs a lazy initialization of a field:
if (field == null) {
field = new ();
}
|
| Fetches the last element of an array:
array[array.length - 1]
|
| Generates a main method declaration
public static void main(String[] args){
}
(The same as |
| Assigns the lesser of the two values to a variable:
= Math.min(, );
|
| Assigns the greater of the two values to a variable:
= Math.max(, );
|
| Inserts |
| Inserts |
| Inserts |
| Inserts |
| Generates a main method declaration
public static void main(String[] args){
}
(The same as |
| Iterates over the elements of an array in the reverse order:
for(int i = array.length - 1; i >= 0; i--) {
= array[i];
}
|
| Surround with
.readLock().lock();
try {
} finally {
.readLock().unlock();
}
See also: WL |
| Inserts |
| Inserts |
| Inserts |
| Inserts |
| Inserts |
| Prints the current class and method to
|
| Prints parameter names and values to
|
| Prints a value to |
| Inserts |
| Inserts |
| Stores the elements of a |
| Surround with
.writeLock().lock();
try {
} finally {
.writeLock().unlock();
}
See also: RL |
Abbreviation | Description | Template text | Can be applied in |
---|---|---|---|
C
| Surround with Callable |
java.util.concurrent.Callable<$RET$> callable = new java.util.concurrent.Callable<$RET$>() {
public $RET$ call() throws Exception {
$SELECTION$
$END$
}
};
| 1 Java statement |
I
| Iterate Iterable or array |
for ($ELEMENT_TYPE$ $VAR$ : $SELECTION$) {
$END$
}
| 1 Java statement |
RL
| Surround with ReadWriteLock.readLock |
$LOCK$.readLock().lock();
try {
$SELECTION$
} finally {
$LOCK$.readLock().unlock();
}
| 1 Java statement |
St
| String |
String
| 1 Java statement Declaration Expression |
WL
| Surround with ReadWriteLock.writeLock |
$LOCK$.writeLock().lock();
try {
$SELECTION$
} finally {
$LOCK$.writeLock().unlock();
}
| 1 Java statement |
else-if
| Add else-if branch |
else if($CONDITION$){
$END$
}
| Else position |
fori
| Create iteration loop |
for(int $INDEX$ = 0; $INDEX$ < $LIMIT$; $INDEX$++) {
$END$
}
| Java statement |
geti
| Inserts singleton method getInstance |
public static $CLASS_NAME$ getInstance() {
return $VALUE$;
}
| 1 Declaration |
ifn
| Inserts 'if null' statement |
if ($VAR$ == null) {
$END$
}
| 1 Java statement |
inn
| Inserts 'if not null' statement |
if ($VAR$ != null) {
$END$
}
| 1 Java statement |
inst
| Checks object type with instanceof and down-casts it |
if ($EXPR$ instanceof $TYPE$) {
$TYPE$ $VAR$ = ($TYPE$)$EXPR$;
$END$
}
| 1 Java statement |
itar
| Iterate elements of array |
for(int $INDEX$ = 0; $INDEX$ < $ARRAY$.length; $INDEX$++) {
$ELEMENT_TYPE$ $VAR$ = $ARRAY$[$INDEX$];
$END$
}
| 1 Java statement |
itco
| Iterate elements of java.util.Collection |
for($ITER_TYPE$ $ITER$ = $COLLECTION$.iterator(); $ITER$.hasNext(); ) {
$ELEMENT_TYPE$ $VAR$ =$CAST$ $ITER$.next();
$END$
}
| 1 Java statement |
iten
| Iterate java.util.Enumeration |
while($ENUM$.hasMoreElements()){
$TYPE$ $VAR$ = $CAST$ $ENUM$.nextElement();
$END$
}
| 1 Java statement |
iter
| Iterate Iterable or array |
for ($ELEMENT_TYPE$ $VAR$ : $ITERABLE_TYPE$) {
$END$
}
| 1 Java statement |
itit
| Iterate java.util.Iterator |
while($ITER$.hasNext()){
$TYPE$ $VAR$ = $CAST$ $ITER$.next();
$END$
}
| 1 Java statement |
itli
| Iterate elements of java.util.List |
for (int $INDEX$ = 0; $INDEX$ < $LIST$.size(); $INDEX$++) {
$ELEMENT_TYPE$ $VAR$ = $CAST$ $LIST$.get($INDEX$);
$END$
}
| 1 Java statement |
itm
| Iterate keys and values of java.util.Map |
for (java.util.Map.Entry<$KEY_TYPE$, $VALUE_TYPE$> $ENTRY$: $MAP$.entrySet()) {
$KEY_TYPE$ $KEY$ = $ENTRY$.getKey();
$VALUE_TYPE$ $VALUE$ = $ENTRY$.getValue();
$END$
}
| Java statement |
ittok
| Iterate tokens from String |
for (java.util.StringTokenizer $TOKENIZER$ = new java.util.StringTokenizer($STRING$); $TOKENIZER$.hasMoreTokens(); ) {
String $VAR$ = $TOKENIZER_COPY$.nextToken();
$END$
}
| 1 Java statement |
lazy
| Performs lazy initialization |
if ($VAR$ == null) {
$VAR$ = new $TYPE$($END$);
}
| 1 Java statement |
lst
| Fetches last element of an array |
$ARRAY$[$ARRAY$.length - 1]
| 1 Expression |
main
| main() method declaration |
public static void main(String[] args){
$END$
}
| Declaration |
mn
| Sets lesser value to a variable |
$VAR$ = Math.min($VAR$, $END$);
| 1 Java statement |
mx
| Sets greater value to a variable |
$VAR$ = Math.max($VAR$, $END$);
| 1 Java statement |
prsf
| private static final |
private static final
| 1 Declaration |
psf
| public static final |
public static final
| 1 Declaration |
psfi
| public static final int |
public static final int
| 1 Declaration |
psfs
| public static final String |
public static final String
| 1 Declaration |
psvm
| main() method declaration |
public static void main(String[] args){
$END$
}
| Declaration |
ritar
| Iterate elements of array in reverse order |
for(int $INDEX$ = $ARRAY$.length - 1; $INDEX$ >= 0; $INDEX$--) {
$ELEMENT_TYPE$ $VAR$ = $ARRAY$[$INDEX$];
$END$
}
| 1 Java statement |
serr
| Prints a string to System.err |
System.err.println($END$);
| 1 Java statement |
serrc
| System.err::println |
System.err::println
| 1 Consumer function |
souf
| Prints a formatted string to System.out |
System.out.printf("$END$");
| 1 Java statement |
sout
| Prints a string to System.out |
System.out.println($END$);
| 1 Java statement |
soutc
| System.out::println |
System.out::println
| 1 Consumer function |
soutm
| Prints current class and method names to System.out |
System.out.println("$CLASS_NAME$.$METHOD_NAME$");
| 1 Java statement |
soutp
| Prints method parameter names and values to System.out |
System.out.println($FORMAT$);
| 1 Java statement |
soutv
| Prints a value to System.out |
System.out.println("$EXPR_COPY$ = " + $EXPR$);
| 1 Java statement |
thr
| throw new |
throw new
| 1 Java statement |
toar
| Stores elements of java.util.Collection into array |
$COLLECTION$.toArray(new $COMPONENT_TYPE$[0])$END$
| 1 Expression |